In an earlier post I showed how to add Mockito to Eclipse target definitions. This allows plug-in developers to write and run Mockito-based unit tests both inside the Eclipse IDE and in Maven Tycho builds.
There was, however, one missing piece to this functionality – the ability to run tests using the Mockito JUnit 5 extension. The specific issue was that the mockito-junit-jupiter
JAR was not structured as a bundle that would work with an OSGi classloader.
In the most recent version of Mockito (3.7) this has now been fixed and Eclipse RCP developers can now begin to use the extension.
Adding Mockito to your Eclipse RCP target platform
In the past, a new version of Mockito (or any third-party library) would be difficult to incorporate into your target platform. Luckily, there has also been a recent change in the m2e PDE tooling that allows us to include Maven artifacts directly.
So instead of waiting for Mockito to be updated in Eclipse Orbit or wrapping it in a custom plug-in, we can now simply add Mockito and its dependencies (Byte Buddy and Objenesis) as Maven target entries.
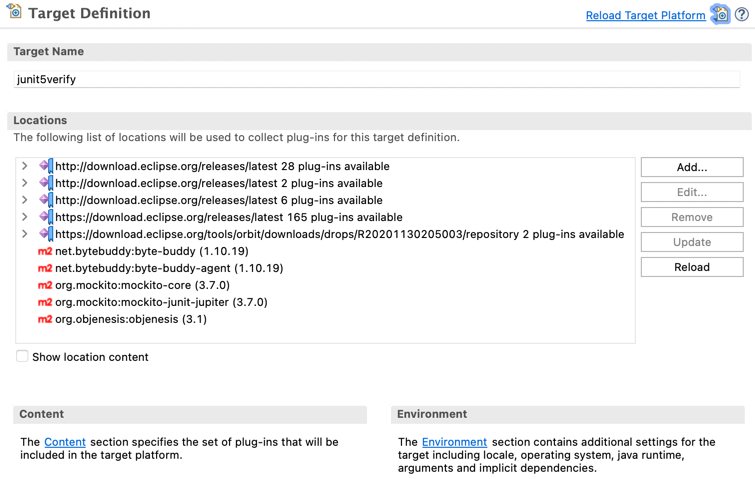
After reloading the target, you can add a dependency in your test fragment manifest to the org.mockito.junit-jupiter
bundle and start writing tests.
@ExtendWith(MockitoExtension.class)
class HelloWorldService_Mockito_ExtensionMock_Test {
@Mock
private HelloWorldService helloWorldService;
@Test
void test() {
when(helloWorldService.sayHello()).thenReturn("Hello!");
assertEquals("Hello!", helloWorldService.sayHello());
}
}
Wrapping up
I’ve updated the projects in the GitHub repository with the new target definition locations and a new test exercising the Mockito JUnit 5 extension.